HackerRank Python : Message Objects Solution
Problem
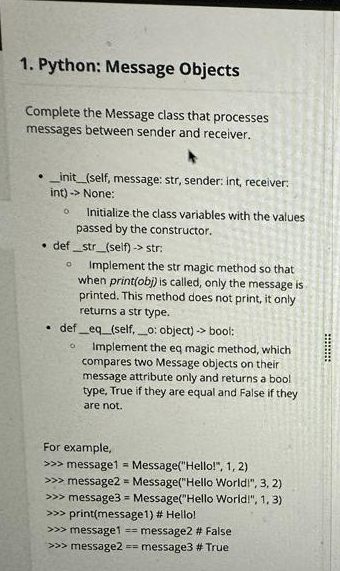
Solution
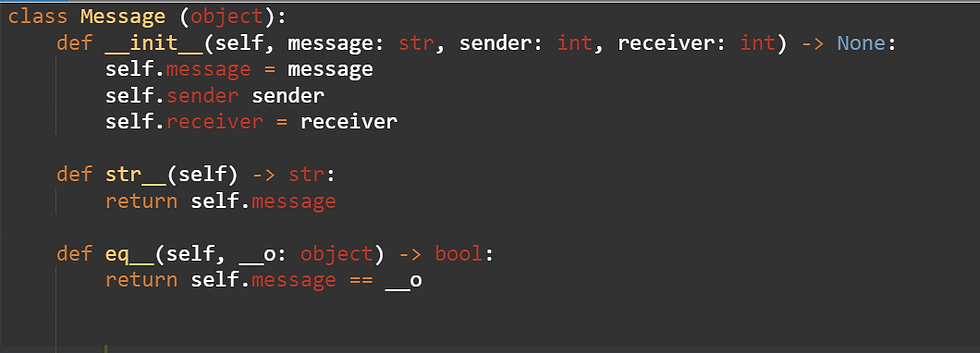
Explanation
This Python code defines a Message class representing a message object with certain properties (message, sender, and receiver) and two special methods (__str__ and __eq__). Here's a detailed explanation of each line:
1. class Message (object): This line defines a new class called Message that inherits from the base class object. The object class is the most basic class in Python from which all other classes are derived. However, in Python 3.x, it is not necessary to explicitly inherit from object as it is the default superclass.
2. def __init__(self, message: str, sender: int, receiver: int) -> None: This line defines the constructor method (__init__) of the Message class. It takes four arguments:
self: A reference to the instance of the class itself.
message: str: The message content, which is expected to be a string.
sender: int: The sender's identifier, expected to be an integer.
receiver: int: The receiver's identifier, expected to be an integer. The -> None part indicates that this method does not return any value.
3. self.message = message: This line assigns the input message to the instance variable self.message.
4. self.sender = sender: There is a syntax error in this line. It should be self.sender = sender. This line assigns the input sender to the instance variable self.sender.
5. self.receiver = receiver: This line assigns the input receiver to the instance variable self.receiver.
6. def str__(self) -> str: This line defines a special method called __str__ for the Message class. The method takes one argument, self, and returns a string. The __str__ method is called by Python's built-in str() function and print() function to convert an object to a human-readable string representation.
7. return self.message: Inside the __str__ method, this line returns the value of the instance variable self.message as the string representation of the Message object.
8. def eq__(self, __o: object) -> bool: This line defines a special method called __eq__ for the Message class. The method takes two arguments:
self: A reference to the instance of the class itself.
__o: object: Another object to compare with the current instance. The -> bool part indicates that this method returns a boolean value. The __eq__ method is called by Python's built-in == operator to compare two objects for equality.
9. return self.message == __o: Inside the __eq__ method, this line checks if the self.message attribute is equal to the other object (__o). However, this comparison is incorrect, as it should compare the message attribute of both objects. The correct line should be return self.message == __o.message with an additional check to ensure that __o is an instance of the Message class, like so:
if isinstance(__o, Message):
return self.message == __o.message
return False
In summary, this code defines a Message class with a constructor to initialize its properties (message, sender, and receiver) and two special methods for string representation and equality comparison.
Comments